How to debug cookies in Rails and how to solve CookieOverflow issue
Using the browser's developer tools, you may debug cookies in Rails. In Google Chrome, you can access the developer tools by right-clicking on any element on the website and selecting "Inspect". Then, navigate to the "Application" tab and click on "Cookies". You may examine all cookies set for the current domain here, including their name, value, domain, and expiration date. You may also use the developer tools to alter the cookies and watch how your application responds. This is handy for evaluating the functioning of your cookies, such as confirming that a user is still logged in after changing the value of their login cookie.
When CookieOverflow
occurs in your Rails application, it is simple to troubleshoot locally. You can scroll down a bit your error page and click Toggle session dump
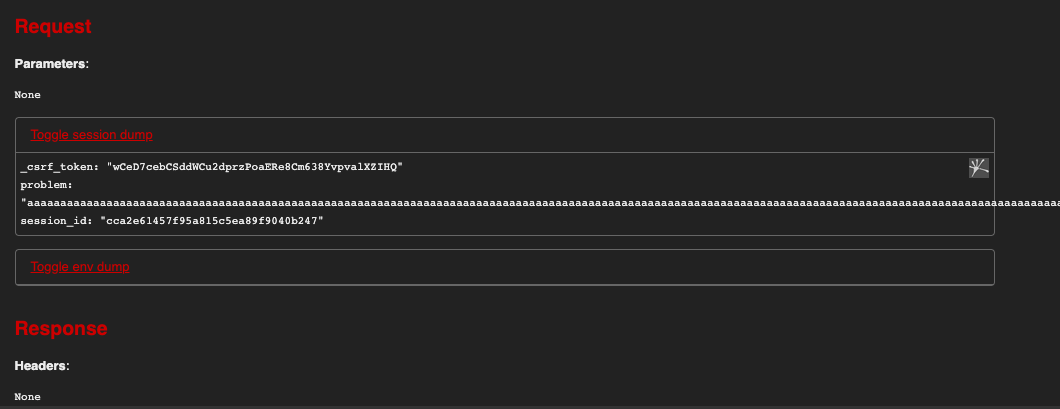
In this example, we see that one of the keys is much larger than it should be. The CookieOverflow
error is raised when the size of the cookie is greater than 4096 bytes. So now you know what the key is and where to find it in the code by name.
What if I can't reproduce the error locally?
It will be difficult to debug in production because you may not have any information other than the ActionDispatch::Cookies::CookieOverflow
error name. That was hard for me to debug, so...
I made a gem cookie_debugger to debug this thing!
# sample implementation of the gem
module MyCookieDebugger
def log_error(request, wrapper)
if wrapper.exception.class == ActionDispatch::Cookies::CookieOverflow
# put information in standard logger(visible in logs file)
# cat log/development.log | grep CookieDebugger
logger(request).public_send(:error, ::CookieDebugger::Debug.call(request))
# or send a message to slack or whatever
# ::Notify::Slack::Channel::Error.call(message: ::CookieDebugger::Debug.call(request))
end
super(request, wrapper)
end
end
ActionDispatch::DebugExceptions.prepend(MyCookieDebugger)
Once we have set this up, we will see a string similar to the one shown below:
CookieDebugger (total size: 17 bytes) from url: http://localhost:3000/ | Cookie: [username=8] | Session: [calculation=4098] [_csrf_token=43] [session_id=32] [session_key_one=3]
In the example above, we can see that the session['calculation']
cost us 4098 bytes as just one key - which is far more than we can handle. So we can now jump into the code and debug who is storing the data in the calculation key.
Happy debugging!